mirror of
https://github.com/filoghost/HolographicDisplays.git
synced 2024-11-22 10:25:41 +01:00
Created Basic tutorial (markdown)
parent
e4522b52c2
commit
ef84193b41
129
Basic-tutorial.md
Normal file
129
Basic-tutorial.md
Normal file
@ -0,0 +1,129 @@
|
||||
# Basic tutorial
|
||||
This tutorial explains how to use the HolographicDisplays API to dynamically create Holograms, as you would do with normal entities. You don't have to use any NMS code, and it's very simple.
|
||||
|
||||
|
||||
## 1) Use the latest version
|
||||
First of all, always use the latest version of HolographicDisplays and ask your plugin's users to do the same. The API is constantly being expanded, and some methods could be missing in previous versions.
|
||||
When using the API, compile your plugin with the latest version, and tell your users to use the **latest version** of HolographicDisplays, because there is no API in some older versions. Some features have also been added during the development, so pay attention to the version you use while coding.
|
||||
|
||||
***
|
||||
|
||||
##2) Check if HolographicDisplays is enabled
|
||||
To use the API, you have to check if the plugin is installed and enabled. You can use the following code in your onEnable() to check that the plugin is installed and is **enabled**.
|
||||
|
||||
|
||||
|
||||
**Add a soft-dependency to your plugin.yml:**
|
||||
```
|
||||
softdepend: [HolographicDisplays]
|
||||
```
|
||||
_If you use `depend` in place of `softdepend` your users will see an exception at server startup if HolographicDisplays is not enabled, and your `onEnable()` will not be called._
|
||||
|
||||
|
||||
**If HolographicDisplays is strictly required**, you can use the following code:
|
||||
```java
|
||||
@Override
|
||||
public void onEnable() {
|
||||
|
||||
if (!Bukkit.getPluginManager().isPluginEnabled("HolographicDisplays")) {
|
||||
getLogger().severe("*** HolographicDisplays is not installed or not enabled. ***");
|
||||
getLogger().severe("*** This plugin will be disabled. ***");
|
||||
this.setEnabled(false);
|
||||
return;
|
||||
}
|
||||
|
||||
// Rest of the code
|
||||
}
|
||||
```
|
||||
|
||||
If it's not strictly required, you can just check if the plugin is enabled, and use a field in your plugin to see if you should make calls to the API:
|
||||
```java
|
||||
private boolean useHolographicDisplays;
|
||||
|
||||
@Override
|
||||
public void onEnable() {
|
||||
useHolographicDisplays = Bukkit.getPluginManager().isPluginEnabled("HolographicDisplays");
|
||||
|
||||
// Rest of the code
|
||||
}
|
||||
```
|
||||
|
||||
***
|
||||
|
||||
##3) Start using the API
|
||||
The API is a static class, called HolographicDisplaysAPI. Through the static method `createHologram(...)` you can create a hologram with some default lines. The method returns the hologram just created at the eye location of a player.
|
||||
Example:
|
||||
```java
|
||||
// Suppose that "player" is a Player object obtained before.
|
||||
Hologram hologram = HolographicDisplaysAPI.createHologram(this, player.getEyeLocation(), "This is a test hologram.");
|
||||
```
|
||||
|
||||
####Static API methods
|
||||
|
||||
`Hologram HolographicDisplaysAPI.createHologram(Plugin owner, Location location, String... lines)`
|
||||
* `owner` - Replace this with your plugin's instance.
|
||||
* `location` - The location where the first line of the hologram will spawn.
|
||||
* `lines` - An array of lines. Can be null.
|
||||
* Returns the new `Hologram` created.
|
||||
|
||||
`Hologram[] HolographicDisplaysAPI.getHolograms(Plugin plugin)`
|
||||
* Returns an array of `Hologram` owned by a given plugin. Please note that this is a copy of the list, and changing this array will have no effect.
|
||||
|
||||
```java
|
||||
// A list of players...
|
||||
Hologram createIndividualHologram(Plugin plugin, Location source, List<Player> whoCanSee, String... lines)
|
||||
// or a single player.
|
||||
Hologram createIndividualHologram(Plugin plugin, Location source, Player whoCanSee, String... lines)
|
||||
```
|
||||
* Just like createHologram(...) but only some players will see it. **IMPORTANT: requires the plugin [ProtocolLib](http://dev.bukkit.org/bukkit-plugins/protocollib/)**.
|
||||
|
||||
####Methods of the class `Hologram` (you can get a `Hologram` with the static API methods)
|
||||
`boolean hologram.update()`
|
||||
* Returns false if the entities fail to spawn.
|
||||
* Should be used after you modify some lines to update the hologram.
|
||||
|
||||
`hologram.hide()`
|
||||
* Hides a hologram, but doesn't delete it.
|
||||
|
||||
`hologram.delete()`
|
||||
* Deletes a hologram permanently, and hides it. Using again update() will throw an exception. After calling this method, you should remove all the references to the hologram.
|
||||
|
||||
`boolean hologram.isDeleted()`
|
||||
* Returns true if the hologram was deleted.
|
||||
|
||||
The following methods can be used to dynamically change the lines. You can't use the placeholders provided by the plugin. Indexes start from 0. List:
|
||||
* `hologram.addLine(String text)`
|
||||
* `hologram.removeLine(int index)`
|
||||
* `hologram.setLine(int index, String text)`
|
||||
* `hologram.insertLine(int beforeIndex, String text);`
|
||||
* `hologram.clearLines();`
|
||||
|
||||
**Note:** if you specify an invalid index, the exception ArrayOutOfBoundsException will be raised.
|
||||
|
||||
`String[] hologram.getLines()`
|
||||
* Returns a copy of the lines of the hologram.
|
||||
|
||||
`int hologram.getLinesLength()`
|
||||
* Returns the amount of lines in the hologram.
|
||||
|
||||
|
||||
Methods relative to the position:
|
||||
|
||||
`hologram.setLocation(Location loc)`
|
||||
* Changes a location of a hologram. You need to call update() after this method. Please do not use it to create moving holograms, a hologram.teleport(...) method will be added.
|
||||
|
||||
Use these methods to get the position and the world of the hologram:
|
||||
* `double hologram.getX()`
|
||||
* `double hologram.getY()`
|
||||
* `double hologram.getZ()`
|
||||
* `World hologram.getWorld()`
|
||||
|
||||
***
|
||||
|
||||
##(Optional) Video tutorial
|
||||
If you still have questions or you need some help, watch this video to learn how to create a plugin with HolographicDisplays, or send me a PM on Bukkit:
|
||||
|
||||
[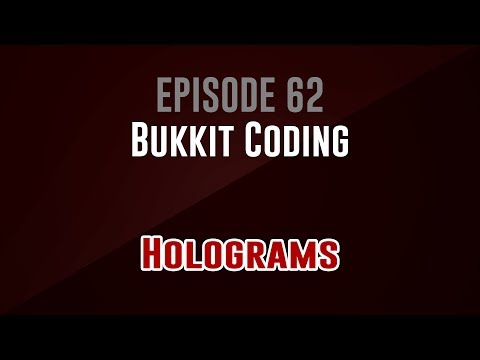](https://www.youtube.com/watch?v=x5jH-8yNRQY)
|
||||
[](https://www.youtube.com/watch?v=5naNP4sZaAk)
|
||||
|
||||
***
|
Loading…
Reference in New Issue
Block a user