mirror of
https://github.com/plan-player-analytics/Plan.git
synced 2025-02-18 21:32:09 +01:00
Updated APIv5 PageExtension API (markdown)
parent
ff40c4f6d0
commit
8ff1e959a9
@ -10,6 +10,7 @@ It consists of these parts:
|
||||
|
||||
- `ResolverService` - For adding HTTP request responses
|
||||
- `ResourceService` - For customizing files and making them customizable by user.
|
||||
- `pageExtensionApi` - Javascript api for extending the React website
|
||||
|
||||
## Table of contents
|
||||
|
||||
@ -21,6 +22,7 @@ It consists of these parts:
|
||||
- [Making resources customizable](#making-resources-customizable)
|
||||
- [Adding javascript to .html resource](#adding-javascript-to-html-resource)
|
||||
- [Adding stylesheet to .html resource](#adding-stylesheet-to-html-resource)
|
||||
- [Javascript API](#javascript-api)
|
||||
|
||||
----
|
||||
|
||||
@ -345,9 +347,8 @@ svc.addScriptsToResource("PluginName", "customized.html", Position.PRE_CONTENT,
|
||||
|
||||
`<script src="./js"></script>` snippets can be added to 3 `Position`s in a .html file:
|
||||
|
||||
- `PRE_CONTENT` - to the `<head>`, loaded before content
|
||||
- `PRE_MAIN_SCRIPT` - before `<script id="mainScript">` (Recommended for libraries and page stucture modifications)
|
||||
- `AFTER_MAIN_SCRIPT` - at the end of the page before `</body>` tag (Recommended for data loading)
|
||||
- `PRE_CONTENT` - to the `<head>`, loaded before content, recommended for stylesheets
|
||||
- `PRE_MAIN_SCRIPT` - Before React script runs, but after pageExtensionApi.js has run, load libraries or call pageExtensionApi
|
||||
|
||||
You can add a query to the URL if your javascript needs parameters, eg `"./some.js?value=some"` and access them with `URIQuery` in `Resolver#resolve`.
|
||||
Some Plan resources use `${placeholder}` replacement - in these cases you can use `"./some.js?player=${playerUUID}"` for example.
|
||||
@ -363,9 +364,8 @@ svc.addStylesToResource("PluginName", "customized.html", Position.PRE_MAIN_SCRIP
|
||||
|
||||
`<link href="./css" rel="stylesheet"></link>` snippets can be added to 3 `Position`s in a .html file:
|
||||
|
||||
- `PRE_CONTENT` - to the `<head>`, loaded before content
|
||||
- `PRE_MAIN_SCRIPT` - before `<script id="mainScript">` (Recommended for libraries)
|
||||
- `AFTER_MAIN_SCRIPT` - at the end of the page before `</body>` tag
|
||||
- `PRE_CONTENT` - to the `<head>`, loaded before content, recommended for stylesheets
|
||||
- `PRE_MAIN_SCRIPT` - Before React script runs, but after pageExtensionApi.js has run
|
||||
|
||||
----
|
||||
|
||||
@ -376,4 +376,47 @@ The PageExtension API will be expanded with javascript methods that allow easier
|
||||
- `callback` : Function (json : Object, error : String)
|
||||
- Makes an xmlhttprequest to the Plan webserver or elsewhere
|
||||
|
||||
Library methods are also available after `PRE_MAIN_SCRIPT` (For example HighCharts APIs) - You might need to check each file you're adding snippets to to be sure.
|
||||
Library methods are also available after `PRE_MAIN_SCRIPT` (For example HighCharts APIs) - You might need to check each file you're adding snippets to to be sure.
|
||||
|
||||
# Javascript API
|
||||
|
||||
There is an example plugin for using the javascript API
|
||||
https://github.com/plan-player-analytics/Example-Plan-API-Plugins/tree/master/PageExtension-API-Example
|
||||
|
||||
Javascript API is defined in https://github.com/plan-player-analytics/Plan/blob/master/Plan/react/dashboard/public/pageExtensionApi.js
|
||||
|
||||
## Methods
|
||||
|
||||
`pageExtensionApi.registerElement('beforeElement', 'card-body-server-as-numbers', render, unmount);`
|
||||
|
||||
Parameter|Accepted values|Description
|
||||
--|--|--
|
||||
0|`'beforeElement'` or `'afterElement'`|Should the element you're rendering be added above or below the element
|
||||
1|`'{id of an element with 'extendable' class}'`|Any element on the page with "extendable" class can be used. If your use-case is not covered, please contact on discord and we'll add more extendable items for it - We didn't add it to everything since there was so much content to go through.
|
||||
2|`async function`|Render function, example: `(context) => {return '<p>Hello world</p>'}`. More about rendering below
|
||||
3|`async function`|Unmount function, in case your code is registering listeners or timers, you can unregister them here.
|
||||
|
||||
### Finding IDs
|
||||
|
||||
Open Browser Dev Tools > Inspector and search for elements with `.extendable` (class)
|
||||
|
||||
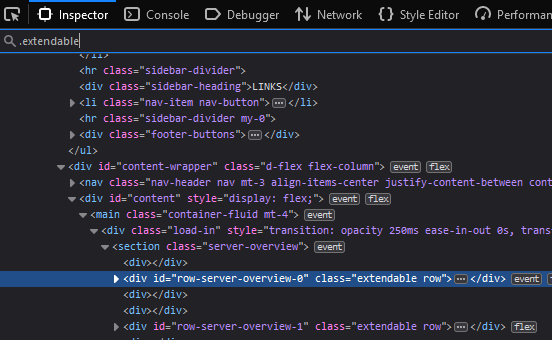
|
||||
|
||||
### Render function
|
||||
|
||||
Render function can use either html elements or html as a String to render elements.
|
||||
|
||||
The `context` contains useful information about the current page, as well as other utilities. `console.log(context)` to see contents
|
||||
|
||||
```js
|
||||
const render = async (context) => {
|
||||
/* Any code that needs to run before the element is added to DOM */
|
||||
return '<p>Hello world</p>';
|
||||
}
|
||||
|
||||
const render = () => {
|
||||
const para = document.createElement("p");
|
||||
para.innerText = "Hello world";
|
||||
return para;
|
||||
}
|
||||
```
|
Loading…
Reference in New Issue
Block a user